Get rid of the ViewHolder, forever!
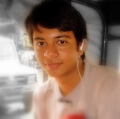
Kirtan Thakkar
Life is all about learningIf you are an android developer, you know how painful it is to code a simple list. You need to write a handful of code just to make a list working without any data in it. An RecyclerView.Adapter
and ViewHolder
if you remember.
Viewholders are used to bind your views once to get rid of the findViewById
calls which reduces performance while scrolling. To get rid of the view holders, you need to write one simple magic line which will work for all your adapters.
For that there are some prerequisites. You must be using:
- Kotlin Language (at least to write that magical view holder)
- DataBinding (It's absolutely magical, trust me!)
- RecyclerView (of course)
Let's write it:
That's it! Yes, that's all. You can use this view holder on every adapter. No need for any changes.
For example, when you have to provide a view holder class to adapter for your item_list.xml
use it something like this:
To access your views, you can use the binding from the onBindViewHolder
method with holder.binding.textView
.
A more detailed example:
Simple, isn't it? #BuildBetterApps