Keep your Firebase Realtime Database connections to minimum
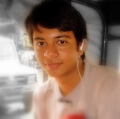
Kirtan Thakkar
Life is all about learningCreating a process creates an persistence connection to the firebase, even if you app is in the background. So, disconnecting that open connection to the database when your app is not in use will save data as well as your precious simultaneous connections if you are on free Spark plan.
For this, to implement on your Android app, we can use the Activity Lifecycle Callbacks and disconnect the connection when the app is in the background.
Look at the below snippet:
This is really simple. So, after your app goes into the background, it will wait for the milliseconds defined in delayedTimeMillis
and after that it will disconnect the connection. If in between that time, your app is again brought back into the foreground, it will not disconnect the connection.
There is also one another very similar approach from the Doug Stevenson here. Whatever you use will do the same thing.
Library Available
Update: I have built a library for simplified background checking. This can be helpful for detecting background state changing. Check out BackgroundManager
So, go ahead, save your connections and save user's data. #BuildBetterApps