Retain precision of double in Java with BigDecimal
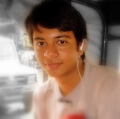
Kirtan Thakkar
Life is all about learningIf you have ever worked with the double
values when you need precision, you might have came across a weird issue where you lost the precision of your value with a very little number. Like, when you add 0.1
3 times you get 0.30000000000000004
instead of 0.3
.
Here are some examples:
5.6
--- 5.8
: 11.399999999999999
0.1
--- 0.1
+ 0.1
: 0.30000000000000004
1
- 0.33
: 0.6699999999999999
(For more details on why this happens, refer to this Stack Overflow answer)
So, Java has a BigDecimal
class which can solve this issue. I have made a small program, from which you can understand how you can get your precision right.
You can run the program and analyze the output after each print statement, to get the better understanding.
Here is the code:
Run your self and play with it for better understanding of when you loose the precision and how to get the correct value before precision is lost.
And Here is the output:
When assigning the double value to BigDecimal
, make sure you have not already lost your precision. Better create BigDecimal
from String
value. If you already have double with lost precision, get it formatted in String with "%.2f"
(for example) before creating BigDecimal
for right value.
It is extremely important to retain the precision when working with the currencies.
Just make sure you don't loose the precision next time.
Happy coding!